Create JSON Web Tokens (JWT) – Using DotNet MVC Core 7.0 – Part 01
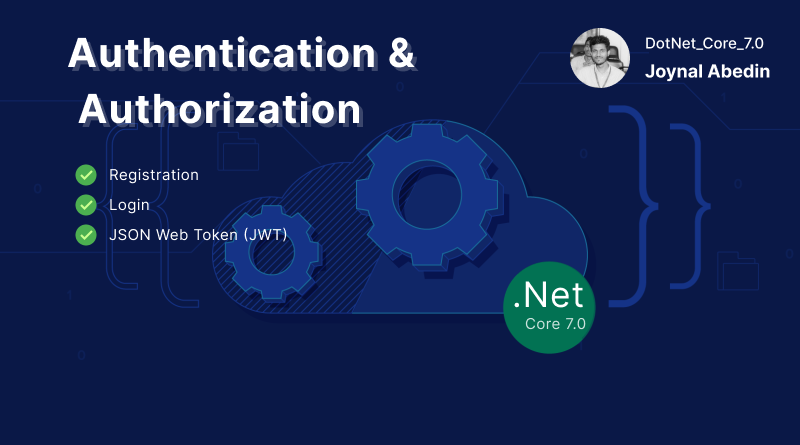
Step_01: First of all create a project with some name & in the project create folder named Models.
Right click project file -> Add-> New Folder-> Folder_Name -> click Enter. Now you can see Models folder in project file.

Step_02: In Models folder we will create two class named User & UserDto.
Dto means Data Transfter Object. This class will be used for create user Registration & Login.
Right click Models folder -> Add-> New Class -> select Empty Class -> Type User class name. Now write some code same as below –
namespace Authentication_AuthorizationAPI.Models
{
public class User
{
public string UserName { get; set; } = string.Empty;
public string PasswordHash { get; set; } = string.Empty;
}
}
Here i assign in User class two properties named UserName & PasswordHash which is initiall declared empty string.
Which way we created User class , the same way we will create UserDto class. And Write this code same as below –
using System;
namespace Authentication_AuthorizationAPI.Models
{
public class UserDto
{
public required string UserName { get; set; }
public required string Password { get; set; }
}
}
Above this UserDto class we assigned two properties required. Here user must be fill this properties otherwise user won’t be able to execution.
Step_03: Create Controller class named AuthController.
Right Click Controller folder -> Add -> New Scaffolding -> Api Controller – Empty -> Next -> Write Controller Name -> Press Enter. You will see same as below –

Step_04: Add Crypt.Net-Next NuGet Package Manager.
Right click Dependencies -> NuGet Package -> search NuGet.Net-Next & install it.
Step_05: Create Register method in AuthController. And write this code same as –
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Authentication_AuthorizationAPI.Models;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
namespace Authentication_AuthorizationAPI.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class AuthController : ControllerBase
{
public static User user = new User();
[HttpPost("register")]
public ActionResult<User> Register(UserDto request)
{
string passwordHash = BCrypt.Net.BCrypt.HashPassword(request.Password);
user.UserName = request.UserName;
user.PasswordHash = passwordHash;
return Ok(user);
}
}
}
Here in Register method is a Post method where i passed UserName & Password which type is UserDto. Here i assign request data in user object. After processing this data return User type of data. If we run this project & pass UserDto types of data then it will return below type of data –


Step_06: Create Login method in AuthController. And write this code same as –
[HttpPost("login")]
public ActionResult<User> Login(UserDto request)
{
if (user.UserName != request.UserName)
{
return BadRequest("User not found");
}
if (!BCrypt.Net.BCrypt.Verify(request.Password, user.PasswordHash))
{
return BadRequest("Wrong password");
}
return Ok(user);
}
Here in Login method is a Post method where i passed UserName & Password which type is UserDto. After processing this data return User type of data. here i tried to match user request data with login data. If it matched with login data then we sent user object otherwise sent wrong message.
Step_07: And Finally Create CreateToken method in AuthController. And write this code same as –
Before writing code, first of all we need to install some Nuget package-
- Microsoft.AspNetCore.Authentication.JwtBearer
- System.IdentifyModel.Token.Jwt
After install this Nuget package we will set Token value in appsettings.json file same as below –

Now implement CreateToken method same as below –
private string CreateToken(User user)
{
List<Claim> claims = new List<Claim>
{
new Claim(ClaimTypes.Name, user.UserName)
};
var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(_configuration.GetSection("AppSettings:Token").Value!));
var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256Signature);
var token = new JwtSecurityToken(
claims: claims,
expires: DateTime.Now.AddDays(1),
signingCredentials: creds
);
var jwt = new JwtSecurityTokenHandler().WriteToken(token);
return jwt;
}
If we summarize the final code. Then it will be same as below-
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Claims;
using System.Text;
using System.Threading.Tasks;
using Authentication_AuthorizationAPI.Models;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.IdentityModel.Tokens;
using System.IdentityModel.Tokens.Jwt;
namespace Authentication_AuthorizationAPI.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class AuthController : ControllerBase
{
public static User user = new User();
private readonly IConfiguration _configuration;
public AuthController(IConfiguration configuration)
{
_configuration = configuration;
}
//Register method
[HttpPost("register")]
public ActionResult<User> Register(UserDto request)
{
string passwordHash = BCrypt.Net.BCrypt.HashPassword(request.Password);
user.UserName = request.UserName;
user.PasswordHash = passwordHash;
return Ok(user);
}
//Login method
[HttpPost("login")]
public ActionResult<User> Login(UserDto request)
{
if (user.UserName != request.UserName)
{
return BadRequest("User not found");
}
if (!BCrypt.Net.BCrypt.Verify(request.Password, user.PasswordHash))
{
return BadRequest("Wrong password");
}
string token = CreateToken(user);
return Ok(token);
}
private string CreateToken(User user)
{
List<Claim> claims = new List<Claim>
{
new Claim(ClaimTypes.Name, user.UserName)
};
var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(_configuration.GetSection("AppSettings:Token").Value!));
var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256Signature);
var token = new JwtSecurityToken(
claims: claims,
expires: DateTime.Now.AddDays(1),
signingCredentials: creds
);
var jwt = new JwtSecurityTokenHandler().WriteToken(token);
return jwt;
}
}
}
If we run this project then continue Register and Login. We will get JWT (Json Web Token).
1.Register:

2. Login:

Push it to the limit cool Wolf you are the best and you can do everything https://www.samsung.com smkmkplobydlmcrjmzgvx
Push it to the limit cool Wolf! You are the best and you can do everything! It’ll all work out very very very soon! https://www.samsung.com smkmkplobydlmcrjmzgvx
Push it to the limit cool Wolf! You are the best and you can do everything! It’ll all work out very very very soon! https://www.samsung.com smkmkplobydlmcrjmzgvx
V74T4YPNNC88 https://dzen.ru sgjvhnbtcbtfgdjgfb
Push it to the limit cool Wolf! You are the best and you can do everything! It’ll all work out very very very soon! https://www.samsung.com smkmkplobydlmcrjmzgvx
От 25 000 рублей с САЙТА и РЕКЛАМНОЙ КАМПАНИИ. Подробнее по ссылке: https://google.com
От 25 000 рублей с САЙТА и РЕКЛАМНОЙ КАМПАНИИ. Подробнее по ссылке: https://google.com
Thank you For your hard work over the years! For this, we give you the opportunity. https://google.com#1234567890 For more information, see the instructions. skfhjvkjsdjsrbhvbsrfhkis
Thank you For your hard work over the years! For this, we give you the opportunity. https://google.com#1234567890 For more information, see the instructions. skfhjvkjsdjsrbhvbsrfhkis
Push it to the limit cool Wolf! You are the best and you can do everything! It’ll all work out very very very soon! https://www.samsung.com smkmkplobydlmcrjmzgvx
Hello! I simply would like to offer you a huge thumbs up for the great information you have right here on this post. I will be coming back to your blog for more soon.
Wow, incredible weblog structure! How long have you ever been blogging for?
you make blogging look easy. The total look of your web site is magnificent,
let alone the content material! You can see similar here najlepszy sklep
Муж на час [url=]https://myzh-na-chas777.ru/[/url] услуга вызвать мужа на час
Hello!
Этот стройматериал легко транспортируется в герметичных пакетах. Перед нанесением смесь необходимо смешать с водой в требуемых пропорциях. Сухую [url=https://gips-shtukatur.blogspot.com/2024/04/fishkaremontaby.html]штукатурку[/url] нужно выбирать исходя из основных характеристик, особенностей применения. [url=http://fishkaremonta.ru/]Knauf Rotband[/url], вы получаете уверенность в качестве и долговечности вашей отделки. Сделайте свой выбор в [url=http://107maek.ru/]пользу[/url] надежных и проверенных [url=https://raskrutitut.ru/]материалов[/url] от Ротбанд!
[url=https://kursorbymarket.nethouse.ru/articles/google]1[/url]
[url=https://sport-i-dieta.blogspot.com/2024/05/40.html]2[/url]
[url=https://kirill-spektor.blogspot.com/2024/05/40.html]3[/url]
[url=https://raskrutitut.blogspot.com/2024/05/blog-post.html]4[/url]
[url=http://belnotebook.www.by/news/23240.html]5[/url]
[url=http://televizor.www.by/news/23241.html]6[/url]
[url=http://germetik-dereva.www.by/news/23242.html]7[/url]
[url=http://kupit-komputer.www.by/news/23243.html]8[/url]
[url=http://noutbuk.www.by/news/23244.html]9[/url]
[url=http://iphone-apple.www.by/news/23245.html]10[/url]
[url=https://kirillproweb.narod.ru/news/razoblachaem_tajny_proizvodstva_gipsovoj_shtukaturki/2024-05-23-25]11[/url]
[url=https://pcmarket-by.ru.gg/Google.htm]12[/url]
[url=https://pcmarket.tilda.ws/]13[/url]
[url=https://www.google.com/search?q=site%3Agips-shtukatur.blogspot.com%2F2024%2F04%2Ffishkaremontaby.html&newwindow=1&sca_esv=245dc9e7f862aecc&hl=en&gbv=2&sxsrf=ADLYWIK9PCWJaJBPQ2S_W-HUtJUKZZkfyA%3A1715929789960&ei=vQJHZqmVOvCRxc8Ps7WR2Ag&ved=0ahUKEwjp59vMkJSGAxXwSPEDHbNaBIsQ4dUDCBA&oq=site%3Agips-shtukatur.blogspot.com%2F2024%2F04%2Ffishkaremontaby.html&gs_lp=Egxnd3Mtd2l6LXNlcnAiPXNpdGU6Z2lwcy1zaHR1a2F0dXIuYmxvZ3Nwb3QuY29tLzIwMjQvMDQvZmlzaGthcmVtb250YWJ5Lmh0bWxIAFAAWABwAHgAkAEAmAEAoAEAqgEAuAEMyAEAmAIAoAIAmAMAkgcAoAcA&sclient=gws-wiz-serp]14[/url]
В профессии использую карты банка Казахстана. Пользуюсь картой Казахстана для оплаты в США, оформил на https://getkz.cards, всё ок.
[url=https://human-design-professional.ru]Консультация Дизайн Человека – human design[/url]
[url=https://dizain-cheloveka-gov.ru]Дизайн Человека подробно[/url]
priligy for sale Hang in there, one day at a time God and the Universe
Free [url=https://cuminporn.com/4k+nude+wallpaper]4k nude wallpapers[/url] and adult images
Hello !
Good evening. A 7 excellent site 1 that I found on the Internet.
Check out this site. There’s a great article there. [url=https://profcerto.com.br/apostas/examinando-as-estrategias-de-marketing-das-casas-de-apostas-online-para-atrair-e-fidelizar-jogadores/]https://profcerto.com.br/apostas/examinando-as-estrategias-de-marketing-das-casas-de-apostas-online-para-atrair-e-fidelizar-jogadores/[/url]
There is sure to be a lot of useful and interesting information for you here.
You’ll find everything you need and more. Feel free to follow the link below.
Octavio xmsWtfYonexNXrDscN 5 20 2022 cytotec over the counter usa
Hello comrades
Hi. A 7 perfect website 1 that I found on the Internet.
Check out this site. There’s a great article there. https://solobelcanto.it/guida-nei-casino/da-cleopatra-a-caesars-palace-lincontro-epico-di-casino-e-storia/
There is sure to be a lot of useful and interesting information for you here.
You’ll find everything you need and more. Feel free to follow the link below.
[url=https://moskva-human-design.ru/]Консультация Дизайн Человека – human design[/url]
[url=https://moskva-human-design.ru/]«Дизайн человека»[/url]
[url=https://moskva-human-design.ru/]Дизайн Человека подробно[/url]
[url=https://moskva-human-design.ru/]Дизайн человека (Human design) – расчет карты онлайн[/url]
[url=https://moskva-human-design.ru/]Дизайн Человека подробно[/url]
[url=https://moskva-human-design.ru/]Дизайн Человека подробно[/url]
[url=https://moskva-human-design.ru/]Дизайн человека (Human design) – расчет карты онлайн[/url]
[url=https://moskva-human-design.ru/]Карта Дизайна Человека или Бодиграф[/url]
[url=https://moskva-human-design.ru/]Консультация Дизайн Человека – human design[/url]
Ищите в гугле
[url=https://piavee-profile.tv53.ru/article?uqsgqr.html]egze[/url]
[url=https://hfyycz-profile.ilyx.ru/article?hkiyti.html]dklc[/url]
[url=https://nnzbxz-profile.ilyx.ru/article?ziprvs.html]rpip[/url]
[url=https://meurvg-profile.ilyx.ru/article?dkhduu.html]rtfl[/url]
m10crs
https://freeporn.town
https://freeporn.town
xEmWvEzL QsUZEo kxdB IzSJhj GdHxVnsu VuOO
u2tr7p
jht XbPbIk CCgjeul
https://tits.town
BwSMk00UoUk4kWoqQpUAzmist
9llltu
https://fanny.world
Hello.
This post was created with XRumer 23 StrongAI.
Good luck 🙂
Hello!
This post was created with XRumer 23 StrongAI.
Good luck 🙂
Espectro de vibracion
Sistemas de calibración: esencial para el rendimiento fluido y productivo de las dispositivos.
En el ámbito de la tecnología contemporánea, donde la productividad y la estabilidad del aparato son de gran significancia, los aparatos de balanceo cumplen un función esencial. Estos equipos especializados están diseñados para ajustar y estabilizar partes rotativas, ya sea en maquinaria industrial, vehículos de desplazamiento o incluso en equipos caseros.
Para los técnicos en reparación de dispositivos y los especialistas, utilizar con dispositivos de equilibrado es fundamental para garantizar el desempeño uniforme y estable de cualquier dispositivo rotativo. Gracias a estas soluciones avanzadas avanzadas, es posible minimizar sustancialmente las oscilaciones, el sonido y la carga sobre los soportes, prolongando la longevidad de piezas caros.
De igual manera significativo es el tarea que cumplen los sistemas de equilibrado en la atención al consumidor. El ayuda especializado y el soporte regular utilizando estos equipos permiten brindar prestaciones de óptima calidad, mejorando la agrado de los consumidores.
Para los titulares de proyectos, la aporte en unidades de ajuste y dispositivos puede ser fundamental para optimizar la rendimiento y eficiencia de sus sistemas. Esto es especialmente importante para los dueños de negocios que manejan reducidas y modestas emprendimientos, donde cada elemento es relevante.
Asimismo, los aparatos de calibración tienen una vasta aplicación en el área de la protección y el gestión de excelencia. Facilitan detectar potenciales errores, previniendo intervenciones onerosas y averías a los equipos. Incluso, los resultados generados de estos equipos pueden usarse para mejorar métodos y incrementar la reconocimiento en plataformas de exploración.
Las zonas de utilización de los dispositivos de balanceo abarcan múltiples sectores, desde la producción de ciclos hasta el monitoreo de la naturaleza. No importa si se refiere de extensas elaboraciones industriales o pequeños establecimientos de uso personal, los equipos de ajuste son esenciales para promover un rendimiento óptimo y sin riesgo de paradas.
o1f8ua
Direct impression can cause harm as well — should you
attempt to raise heavy weights with a jerking movement,
you could tear the tendons. A good hypertrophy coaching routine ought to already embrace some bench urgent
and overhead urgent, which is nice for increase our front delts.
And it ought to embrace some chin-ups and rows, that are nice for build
up our rear delts. To build broader shoulders, although, we have to construct greater facet delts.
Sadly, the large compound lifts aren’t always the best for bulking up our side delts,
and they also often lag behind. This compound shoulder exercise entails pressing a barbell mounted in a
landmine, giving the bar a set bar path as you press
up at a diagonal angle.
We additionally describe house treatments and
treatments that can present reduction. The supraspinatus may be tested by having the affected person abduct the shoulders to ninety degrees in ahead
flexion with the thumbs pointing downward. The patient
then makes an attempt to raise the arms against examiner resistance (Figure 3).
Lean ahead till your higher body is barely above parallel to the ground, along with your upper arms hanging
straight down. Standing extra upright transfers more
of the work to your traps and higher back, however leaning ahead
like this makes for a incredible mass builder in your
complete back. Targeting shoulder ache requires workouts that primarily concentrate on the rotator cuff
muscular tissues, which embody the supraspinatus, infraspinatus, teres minor,
and subscapularis.
As Quickly As the situation, high quality, radiation, and aggravating and
relieving factors of the shoulder pain have been established, the potential of referred pain ought to be excluded.
Neck pain and pain that radiates under the elbow are sometimes subtle indicators of a cervical spine dysfunction that’s mistaken for a shoulder downside.
In this video, John Meadows of Mountain Canine Food
Regimen demonstrates the method to perform incline dumbbell rows.
Whereas not important, it’s a great way to increase your body temperature and increase your heart fee.
You’re not going for cardio health here, simply warming up, so maintain your exercise intensity
low to reasonable. The following is a listing of 5 of the most effective shoulder workout routines for males.
Individuals working in occupations that require a lot of lifting
may also be more more probably to experience shoulder impingement.
Diagnosing shoulder impingement early on is important, as remedy can help forestall
signs from getting worse. People with shoulder impingement usually experience common stiffness and throbbing in the shoulder.
This sort of ache may resemble that of a toothache, quite than the tearing pain of an injured muscle.
Push-ups and bench presses are more challenging on the backside of the range
of movement, so I suspect they rival the overhead press for building bigger entrance delts.
This is dependent upon what quantity of shoulder exercises you
might be doing per session. In Accordance to studies, it is the complete weekly training quantity, rather than the workout frequency, that matters most for muscle development
[2].
Your therapist may additionally use other treatments corresponding to ice, warmth, or
handbook remedy. After a reduction, the affected arm will remain in a sling for a number of weeks to allow the shoulder to recuperate.
Ice the affected shoulder three or four times a day to deal
with soreness. Once the ache and swelling subside,
a bodily therapist can show you workouts to strengthen the shoulder muscle tissue and rebuild your range of
movement.
Some folks can solely bring the barbell to
shoulder height, and that’s nice, but many individuals can go a bit higher.
With a little bit of experimentation, most of us can discover a method to make upright rows really feel great on our shoulder joints and muscle tissue.
When you think shoulders, you probably envision your
deltoid muscles, or the triangular-shaped muscle group
that wraps across the tops of your arm.
Its primary unique perform is to facilitate shoulder flexion, which is when the arm strikes upward in front of your physique, like
during front raises. This shoulder exercise routine makes use of heavy hundreds,
high reps, and compound and isolation exercises to hit every variable.
This painful condition means you have irritation in your upper biceps tendon.
If your shoulders merely crack or pop often without causing you a great deal of discomfort, you may want
to attempt treating your crepitus at house. A benign progress in your shoulder, scapula,
or rib cage known as an osteochondroma can cause your shoulder to crack whenever you elevate your arm.
However if cracking is painful and occurs after a recent injury, there
could probably be an internal muscle pressure, tear, or fracture
that a healthcare skilled needs to deal with. In most instances the surgeon will
take away part of the acromion bone and sometimes part of
the subacromial bursa. If they find another issues
such as arthritis or a rotator cuff tear, they
will tackle those as properly.
The ball-and-socket joint lets you transfer with a 360-degree range of movement, but could be delicate when in comparison with different
joints (hence the issues about coaching volume). There’s a broad choice of shoulder
workout routines, each of which delivers completely different
outcomes and targets completely different muscles.
Constructing upper physique muscle mass and stability is an effective
general ambition to have when figuring out, and the shoulder press contributes to this improvement.
The scapular wall slide is an effective bodyweight train for shoulder mobility and strengthening.
Nevertheless, they’re not the only workout routines you can use for
shoulder mobility. Below is an inventory of alternatives you’ll have the ability to add
to your bag of exercises. However, if you’re on the lookout for
more resistance, you can incorporate resistance bands or free weights like a
pair of dumbbells and a barbell. If you’re actually making an attempt to deliver up your shoulders, do it very first thing every week on Monday whilst you’re fresh from a day with no work.
Barbell presses should be the mainstay of your shoulder programming and can help you construct wholesome shoulders over time.
This is one other of those uncommon exercises that you could train multiple instances in every week, as a
result of again, you are constructing shoulder stability.
You Are additionally doing more than focusing on your delts, training your mid-back muscular tissues too.
In Contrast To standing overhead presses,
the seated variation puts less stress in your lower
back, making them ideal for should you wrestle with lower back issues.
This preparation could be the Magellan Shoulder Series I show on this video.
This collection approaches shoulder integrity from the perspective that scapular movement is
the “foundation” of any urgent exercising. Low reps are a should with
the BUP, because the CNS fatigue from the excessive grip demand is
intense. 2-3 units of 3-5 reps seem to work finest prior to your limit presses for the
day. What we’d like is a drill that provides us the identical feel because the heavy press and the identical effort—all without putting the same strain on the AC joint.
Interesting in that I have used it as a one-size-fits-all
resolution for a selection of issues with the press. A strain can abruptly
result from heavy lifting or an accident, similar to a trip or fall.
That means you’ll nonetheless be your strongest when doing delt exercises although
you’ve already educated your again. Evaluate that to the popular chest+shoulder
exercise routine the place your shoulders are heavily concerned whenever you practice your chest and tired when it’s time to hit
them. A shoulder superset exercise is a high-intensity routine involving two or extra consecutive workout routines that activate the identical shoulder
muscle groups. Shoulder superset workouts goal the three main muscle tissue of the shoulder—the deltoid, trapezius,
and rotator cuff.
Ongoing physical therapy and adherence to skilled recommendation can help keep shoulder
health and ensure long-term success in powerlifting.
Aggressive weightlifting can lead to eccentric posterior glenoid put on and posterior humeral head subluxation, that are common challenges post-surgery.
Addressing posterior glenoid wear is particularly important for patients who were beforehand lively weightlifters.
Apparently, more than 70% of sufferers can count
on to return to sports after complete shoulder arthroplasty.
Always permit for enough relaxation and recovery between sessions to prevent accidents throughout
your overhead press. To forestall this harm, use lower resistance exercises
with extra repetitions to gradually strengthen the rotator cuff muscular tissues.
Stability these exercises with arm raises and external rotations to build up your deltoids
— try the side-lying external rotation earlier than your next exercise.
End your training session with cold compresses to reduce
irritation, and remember to relaxation between gym days.
Get ready for a mixture of compound exercises with heavy weights and
isolation workouts utilizing relatively mild weights the place you chase the
pump. For athletic functions, the significance of strong shoulders can’t be overstated.
Every Time you need upper-body performance, likelihood is you rely on your shoulder power to back you up.
Before training with this plank variation, you should know how to do the extra
fundamental planks. Several different muscles
are additionally addressed, including the glutes and hamstring.
Strengthening the rotator cuff contributes to raised total shoulder mechanics and performance.
Returning to the beginning place involves decreasing the dumbbells whereas turning your palms in order that they face your body once more.
However, you might require 20 or extra weekly sets if you’re a complicated bodybuilder
or lifter. If that describes you, increasing the frequency of your shoulder and
arm workout to twice every week can improve your gains.
In this model of the rear fly, the ball offers you assist whereas also adding a little instability.
The elbows are bent here, so you’re squeezing the shoulder
blades and dealing the shoulders in addition to the upper again. Constructing stronger, fuller shoulders requires an understanding
of the deltoid muscle’s anatomy. This muscle includes three heads – anterior, lateral, and posterior
– each needing particular workouts for balanced improvement.
“For beginners, or anybody that struggles with overhead ROM, I would advocate standing up in opposition to a flat sturdy wall,” he says.
This Is one other move that’s as much about shoulder well being
as it is about shoulder energy.
After that, we’ll keep you updated on the most important
muscle-building research, lifting methods, and workout routines.
There’s an additional set on some workouts, and we’ve
added the incline bench press. Most individuals
don’t need this much quantity, however you would possibly profit from
it. Earlier Than we delve into our shoulder workout, we need to understand exactly what the
shoulder muscle tissue are. In fact, to essentially build sculpted shoulders, you have to hit all the most important muscle tissue in the shoulder joint (yes, there are multiple) — and from
totally different angles, too. Smith machine seated shoulder press has been the favorite shoulder train with
bodybuilders as it builds muscle fast and is a power move.
Since you’re working your rear deltoid with the cable delt row and not your bigger
latissimus dorsi, use a lighter weight than you’d with
the wide-grip cable row.
A better method of gauging progress in the short time period is to see if you’re getting somewhat bit stronger from exercise to exercise, adding reps
or weight to your lateral raises. The upright row works
the forearms, higher arms (elbow flexors), upper back
(traps), and shoulders (side and rear delts).
The limiting issue is commonly our side delts or traps, and so they
are inclined to get one of the best development stimulus.
Here is my web-site: Dball Steroids
BwSMk00UoUk4kWoqQpUAzmist
gf7vaa
bw2mve