Data Transfer iPhone To Watch using SwiftUI
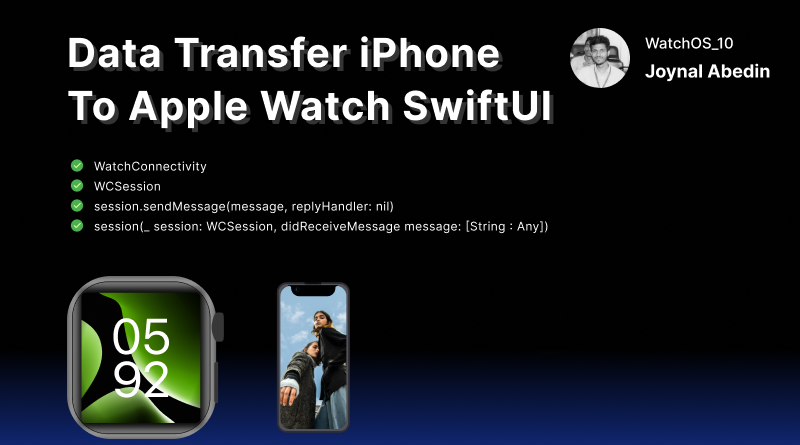
Connecting your Phone to your Watch to send data has never been easier! Follow this easy step-by-step guide, to discover how to pair them as well as what are the most common errors you might encounter.
For the porpouse of the article we will use the sendMessage(_:replyHandler:errorHandler:) method, which works also on the Simulator.
First of all create a project in Xcode that has both the iOS app and the Watch app
Step_01: Click Xcode then choose Create New Project...

Step_02: Then select from WatchOS segment App. If you are already running Xcode, then choose File->New->Project. Then click next.

Step_03: Now give your App name select team & also give Organization Identifier. Finally select Watch App with new Companion iOS app then click Next.

iPhone Section
Step_04: Select ContentView from iphone section & refactor it by PhoneView. Add PhoneVM swift file and write this below code in PhoneVM file:
import Foundation
import UIKit
import SwiftUI
import WatchConnectivity
class PhoneVM: NSObject, ObservableObject {
private let session: WCSession
init(session: WCSession = .default){
self.session = session
super.init()
session.delegate = self
session.activate()
#if os(iOS)
print("Connection provider initialized on phone")
#endif
#if os(watchOS)
print("Connection provider initialized on watch")
#endif
self.connect()
}
func connect(){
guard WCSession.isSupported() else {
print("WCSession not supported")
return
}
session.activate()
}
}
extension PhoneVM: WCSessionDelegate {
func send(message: [String: Any]) -> Void {
session.sendMessage(message, replyHandler: nil) { error in
print(error.localizedDescription)
}
}
func session(_ session: WCSession, activationDidCompleteWith activationState: WCSessionActivationState, error: Error?) {
if let error {
print("session activation failed with error: \(error.localizedDescription)")
}
}
#if os(iOS)
func sessionDidBecomeInactive(_ session: WCSession) {
session.activate()
}
func sessionDidDeactivate(_ session: WCSession) {
session.activate()
}
#endif
}
Here, first of all i imported WatchConnectivity framework. Then i initialize WCSession where i tried to activate this session. When only WCSession.isSupported() is true then this session will be activated. In our PhoneVM extension we implemented WCSessionDelegate & its protocols. In send(message: [String: Any]) function i tried to sent data to watch. Here, i didn’t acceped reply, so that i sent this parameter nil. Remember that data transfer will be only Dictionaries way i means [String: Any] this way.
Step_05: In PhoneView implemented this below code:
import SwiftUI
struct PhoneView: View {
var vm = PhoneVM()
var body: some View {
VStack{
Text("Sent Data To Watch")
.onTapGesture {
vm.send(message: ["iPhone": "Hey Joynal Vai"])
}
}
}
}
#Preview {
PhoneView()
}
In PhoneView i created object of PhoneVM class. Using vm object i called send(message: [String: Any]) method & passed data to watch. When user will click “Sent Data To Watch” then this given data will be sent to watch.
Watch Section:
Step_06: Above this same way we will create WatchVM swift file & refactor ContentView to WatchView. Add this below code in WatchVM file.
import Foundation
import UIKit
import SwiftUI
import WatchConnectivity
class WatchVM: NSObject, ObservableObject {
@Published var getDataFromPhone = ""
private let session: WCSession
init(session: WCSession = .default){
self.session = session
super.init()
session.delegate = self
session.activate()
#if os(iOS)
print("Connection provider initialized on phone")
#endif
#if os(watchOS)
print("Connection provider initialized on watch")
#endif
self.connect()
}
func connect(){
guard WCSession.isSupported() else {
print("WCSession not supported")
return
}
session.activate()
}
}
extension WatchVM: WCSessionDelegate {
func session(_ session: WCSession, activationDidCompleteWith activationState: WCSessionActivationState, error: Error?) {
if let error {
print("session activation failed with error: \(error.localizedDescription)")
}
}
func session(_ session: WCSession, didReceiveMessage message: [String : Any]) {
if let value = message["iPhone"] as? String {
self.getDataFromPhone = value
}
}
}
The same way of PhoneVM here i imported WatchConnectivity framework & activated WCSession. Only one extra method i called here named session(_ session: WCSession, didReceiveMessage message: [String : Any]). I received value Dictionary way & assign this value above declare the variable in WatchVM.
Step_07: Now it’s hight time to show data in Watch. Write this below code in WatchView:
import SwiftUI
struct WatchView: View {
@StateObject private var vm = WatchVM()
var body: some View {
VStack {
Image(systemName: "globe")
.imageScale(.large)
.foregroundStyle(.tint)
Text("Loading Data...")
Text(vm.getDataFromPhone)
}
.padding()
}
}
#Preview {
WatchView()
}
Here, i created object of WatchVM & get data from getDataFromPhone variable & assign it to Text() view.
Step_08: Pair your iPhone & Apple Watch & select iPhoneToWatchDT App Watch App. Run your application & you will see this output:

Pingback: cialis price walmart
Pingback: cialis alternative
Pingback: cialis no prescription overnight shipping
Pingback: online pharmacy no prescription needed lortab
Pingback: dexamethasone online pharmacy
Pingback: viagra generic without prescription
Pingback: tadalafil 20mg how long before sex
Pingback: safe buy viagra online
Pingback: cialis professional 20 lowest price
Pingback: cialis ordering australia
Pingback: effexor pharmacy assistance
Pingback: viagra 50mg price in india online
Pingback: sildenafil tablets in india
Pingback: viagra tablets price in uk
Pingback: generic viagra online europe
Pingback: 50mg viagra
Pingback: prescription viagra usa
Pingback: buy cialisonline
Pingback: cialis 25mg
Pingback: buying cialis from canada
Pingback: canadian online pharmacy cialis
Pingback: metronidazole youtube
Pingback: neurontin dental
Pingback: bactrim g6pd
Pingback: is pregabalin addictive
Pingback: valacyclovir equine
Pingback: nolvadex anabolic
Pingback: metformin physiology
Pingback: lasix principio
Pingback: lisinopril fibrillation
Pingback: groupon semaglutide
Pingback: cost of semaglutide
Pingback: januvia and rybelsus together
Pingback: cephalexin (keflex) 500 mg capsule
Pingback: flagyl 400mg
Pingback: zoloft vs.wellbutrin
Pingback: cephalexin used for ear infections
Pingback: metabolic encephalopathy fibrosis score 0.69 liver escitalopram oxalate 10 mg tablet
Pingback: fluoxetine and alcohol
Pingback: duloxetine hcl dr 60 mg cap price
Pingback: viagra cost in australia
Pingback: does cymbalta help nerve pain
Pingback: reddit lexapro
Pingback: co-gabapentin 100mg
Pingback: ciprofloxacin dosage for uti in adults
Pingback: what is cephalexin used for
Pingback: bactrim for e coli uti
Pingback: bactrim dose for uti 3 days
Pingback: otc amoxicillin
Pingback: side effects of cozaar 50 mg
Pingback: can augmentin be crushed
Pingback: diltiazem package insert
Pingback: price flomax walmart
Pingback: how does depakote work
Pingback: flexeril mechanism of action
Pingback: citalopram hydrobromide 20 mg
Pingback: diclofenac topical gel
Pingback: contrave what is it
Pingback: effexor vs pristiq
Pingback: ezetimibe outcomes trial
Pingback: high dose ddavp
Pingback: allopurinol generic name
Pingback: brand name for aripiprazole
Pingback: amitriptyline 25 mg
Pingback: aspirin vs acetaminophen
Pingback: augmentin amoxicillin
Pingback: ashwagandha breastfeeding
Pingback: bupropion side effects
Pingback: how long for celebrex to work
Pingback: baclofen drug interactions
Have you ever considered writing an ebook or guest authoring on other blogs?
I have a blog centered on the same ideas you discuss and would love to have
you share some stories/information. I know my subscribers would appreciate your work.
If you’re even remotely interested, feel free to send me an e mail.
Tremendous things here. I’m very happy to look your post.
Thanks so much and I’m looking ahead to contact you.
Will you please drop me a mail?
Undeniably believe that which you stated. Your favorite justification appeared to be on the internet the simplest thing
to be aware of. I say to you, I certainly get irked while people consider worries that they just don’t know
about. You managed to hit the nail upon the top and defined
out the whole thing without having side-effects , people
can take a signal. Will probably be back to get more.
Thanks
Pingback: buy semaglutide online
Pingback: remeron for appetite stimulant
Pingback: actos absorption
Pingback: what is robaxin prescribed for
Pingback: best time of day to take abilify
Pingback: acarbose medsafe
Pingback: what are the side effects of protonix
Pingback: repaglinide absorption site
Wow, wonderful blog layout! How lengthy have you been blogging for?
you make running a blog look easy. The full look of your website is great, as smartly as the content material!
You can see similar here najlepszy sklep
Pingback: tizanidine liver
Pingback: l-thyroxine(synthroid)tab 75mcg
Pingback: what are the side effects of venlafaxine
Pingback: sitagliptin side effects pancreatitis
Pingback: stromectol for sale
Pingback: tretinoin and spironolactone
Pingback: interactions for voltaren
Pingback: tamsulosin shrink prostate
Pingback: tadalafil mechanism of action
Pingback: cialis online pills
Pingback: unicare pharmacy vardenafil
Pingback: sildenafil 60 mg reviews
Pingback: levitra online pharmacy
Pingback: brand levitra online pharmacy
Pingback: best pharmacy to get phentermine
Pingback: is sildenafil covered by insurance
Pingback: ivermectin 0.1 uk
Pingback: price of stromectol
Pingback: buy viagra canadian pharmacy
Pingback: is tadalafil available in generic form
Pingback: stromectol order online
Pingback: tadalafil troche cost
Pingback: average price of 100mg viagra
Pingback: vardenafil 75mg
Pingback: ivermectin new zealand
Pingback: where can i buy oral ivermectin
Pingback: vardenafil generic alternative
Pingback: generic ivermectin for humans
Want to improve your SEO rankings and save time? Our premium databases for XRumer and GSA Search Engine Ranker are just what you need!
What do our databases include?
• Active links: Get access to constantly updated lists of active links from profiles, posts, forums, guestbooks, blogs, and more. No more wasting time on dead links!
• Verified and identified links: Our premium databases for GSA Search Engine Ranker include verified and identified links, categorized by search engines. This means you get the highest quality links that will help you rank higher.
• Monthly updates: All of our databases are updated monthly to ensure you have the most fresh and effective links.
Choose the right option for you:
• XRumer premium database:
o Premium database with free updates: $119
o Premium database without updates: $38
• Fresh XRumer Database:
o Fresh database with free updates: $94
o Fresh database without updates: $25
• GSA Search Engine Ranker Verified Links:
o GSA Search Engine Ranker activation key: $65 (includes database)
o Fresh database with free updates: $119
o Fresh database without updates: $38
Don’t waste time on outdated or inactive links. Invest in our premium databases and start seeing results today!
Order now!
P.S. By purchasing GSA Search Engine Ranker from us, you get a high-quality product at a competitive price. Save your resources and start improving your SEO rankings today!
To contact us, write to telegram https://t.me/DropDeadStudio
qui perspiciatis numquam repudiandae omnis sed. cum provident ut voluptatem rerum.
Pingback: keflex ear infection
Pingback: ciprofloxacin hcl 500 mg para que sirve
Pingback: provigil brain pill
Pingback: pregabalin other names
Pingback: how long for lisinopril to work
Pingback: ampicillin stock 50mg/ml
Pingback: cephalexin 500 mg
Pingback: amoxicillin and birth control
Pingback: metformin lactic acidosis symptoms
Pingback: trazodone 150 mg
Pingback: doxycycline dairy
Pingback: valacyclovir para que sirve
It’s awesome in support of me to have a web site, which is beneficial designed
for my knowledge. thanks admin
voluptas repudiandae velit et maxime vero illo rerum sed magnam voluptates ea. dolore saepe voluptatem numquam assumenda voluptatem. aut repellendus omnis illum saepe consequatur cupiditate quia perspiciatis et a totam.
Pingback: chance of breast cancer recurrence without tamoxifen
Pingback: prednisone for ear infection